Error Messages and Developing for BloodHound
This page provides a list of some coding issues a 3rd party indicator may have, in turn causing errors with BloodHound or BlackBird. NinjaTrader is forgiving when running an indicator on a chart, but is strict when running an indicator inside another indicator (i.e. using an indicator in BloodHound).
Error Messages from BloodHound
MaximumBarsLookBack.TwoHundredFiftySix
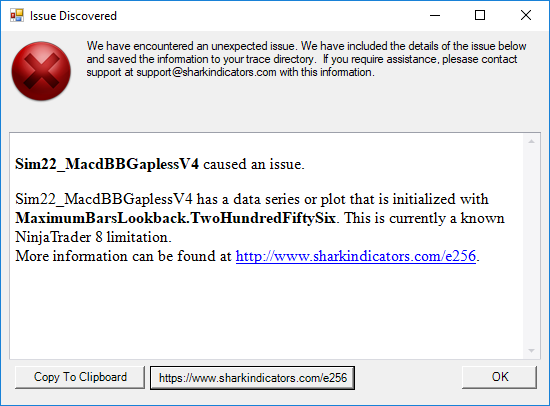
If you recieved this error, an indicator you are using has the MaximumBarsLookBack setting internally locked. NinjaTrader 8 throws an error when validating that indicator's historical data. The setting is MaximumBarsLookBack.TwoHundredFiftySix. NinjaTrader documentation link.
Ask the programmer to remove all references to MaximumBarsLookBack.TwoHundredFiftySix, or change all MaximumBarsLookBack.TwoHundredFiftySix to MaximumBarsLookBack.Infinite Either solution will remove the limitation and error.
// SEARCH FOR ALL INSTANCES OF THIS TEXT //
MaximumBarsLookBack.TwoHundredFiftySix;
// CHANGE THEM TO //
MaximumBarsLookBack.Infinite;
This is a NinjaTrader 8 issue. When indicator data is being validated, using .IsValidDataPoint(x), NinjaTrader 8 throws an error instead of stating the data is invalid. NT 7 did not have this issue.
Other Error Messages
DevExpress... is not a Visual or Visual3D
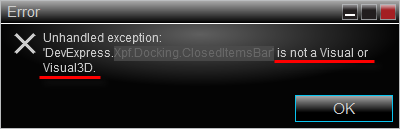
This error is benign. It is caused by a NinjaScript Add On that summons a list of objects and invokes those elements as a Visual object.
The NinjaAddons.ShortcutToolbar Add On is known to cause this error.
Example of offending code
VirtualizingStackPanel verticalStackPanel = null;
foreach (var v in chartGrid.Children)
if ( v is VirtualizingStackPanel &&
// VirtualizingStackPanel is a Visual element
(v as VirtualizingStackPanel).Uid == "ShortCutToolbar")
{
verticalStackPanel = v as VirtualizingStackPanel;
break;
}
Developing for BloodHound
NT 8 Common NinjaScript Mistakes
BloodHound does not have any special requirements to be able to read/use other indicators, except for the MaximumBarsLookBack property as mentioned above.
The list below are common coding mistakes and bad practices that can cause errors.
AddDataSeries()
Brushes
Be sure to follow NinjaTrader's guild lines, Working with Brushes, when defining custom Brushes. The primary concern is making sure custom Brushes are frozen to prevent threading errors, as NinjaTrader's documentation warns about many times.
Side note: Brushes are not used by guest indicators, because they obviously can not draw on a chart, therefore BloodHound & BlackBird ignore Brushes properties.
Do Not modify Brush properties during State.SetDefaults or State.Configure. Doing so causes threading issues for the hosting indicator/strategy (aka BloodHound / BlackBird). Modify Brush properties during State.DataLoaded or afterwards.
Do not modify the Opacity during those States.
else if (State == State.Configure)
Plots
Be sure to initialize Plots in accordance with NinjaTrader requirements. We have seen many indicators initialize Plots in the wrong OnStateChange() state.
See documentation here, Indicator » AddPlot().
Follow the rules for setting plot values as outlined in the section Setting Values below.
Series<T> Initializing and Synchronizing
BloodHound can read the following Series<T> types: Bool, Int, Double, and Long.
A Series<T> can cause the following errors and other similar errors;
System.IndexOutOfRangeException:
System.ArgumentOutOfRangeException:
System.NullReferenceException:
When calling an indicator from another indicator (i.e. using an indicator inside BloodHound) strict NinjaScript protocols must be followed. The following guidelines can eliminate those errors.
Initializing
All Series<T> must be initialize in the OnStateChanged() » State.DataLoaded section of your code, in accordance with Series<T> NinjaScript documentation. Initializing anywhere else can cause errors when BloodHound tries to access the Series.
Synchronizing
Do not synchronize a Series<T> to a secondary Bars series.
E.G. myData = new Series<double>(this, BarArray[1], MaximumBarsLookBack.Infinite);
Do not specify a BarsArray. Allow the Series to sync to the primary Bars series.
Setting Values
BarsInProgress: If the indicator uses multiple Bars series (multi-timeframes), only set values to the Series during BarsInProgress zero. Setting values in any other Bars series can cause System.ArgumentOutOfRangeException: errors.
Example
protected override void OnBarUpdate()
{
if(BarsInProgress == 0)
{
// Set values during this Bars series
mySeries[0] = Close[0];
}
}
OnBarUpdate(): A public Series<T> must be set in OnBarUpdate(). Setting a public Series<> in other methods may create a synchronization issue. Per NinjaTrader's best practices, BloodHound calls guest indicator data during the OnBarUpdate() event. This is a timing issue with NinjaTrader's event driven methods.
OnMarketData(): A public Series<T> should not be set in this method. BloodHound uses OnBarUpdate() to access indicator values. Guest indicator that set a public Series<> in OnMarketData() return a null value. Values gained from OnMarketData() should be stored and set to public Series<> in OnBarUpdate().
Calculations in OnRender()
NinjaTrader does not call the OnRender() method when an indicator is called by another indicator, as is the case when using an indicator in BloodHound. OnRender() is only called when the indicator is running on a chart. Make sure no data is calculated in this method that is used for Plot values or Series<T> values. Therefore, perform calculations for visual purposes only in this method.
ChartControl & Other Null Objects
ChartControl
This object is Null when an indicator is called by another indicator, as is the case when using an indicator in BloodHound. Make sure ChartControl properties are not used or called when your indicator is running as a guest indicator.
Instrument
This object is Null during State.Configure. Therefore, using the Instrument object directly in a AddDataSeries() will cause a Null reference.
e.g. AddDataSeries( Instrument.FullName, _barsPeriodType, _Period); will throw a Null error, because Instrument is still null during this State.
Please Note:
Other NinjaScript objects may be Null, as well, when an indicator is running inside another indicator. Double check the NinjaScript documentation. NT keeps improving and adding to their documentation all the time.
Manual Removal &
Fixing Developer Extension Errors
These instructions will remove the SharkIndicators software from NinjaTrader. Those needing to remove Dev. Ext. pay attention to steps 5.1 and 6.
-
- Remove all custom NinjaScript code that references BloodHound. Files such as 'TDU Bloodhound Statistics' or 'Smart TradeMarkers'. All custom strategies and indicators that references or uses BloodHound must be removed/uninstalled from NinjaTrader first.
- Protected indicators from 3rd party vendors can be removed by opening NinjaTrader Control Center » Tools menu » 'Remove NinjaScript Assembly'.
- Open source custom indicators and/or strategies will need to be removed manually using 'Windows File Explorer'.
- Close NinjaTrader.
- Uninstall the SharkIndicators software.
- Open the Windows Settings(Win 10) or Windows Control Panel(Win 7).
- Open ‘Apps’ (Win 10) or ‘Programs and Features’ (Win 7).
- Locate and select SharkIndicator, then click Uninstall.
- Restart your computer. This helps remove possible file locks, and has other benefits.
(Do Not start NinjaTrader yet.) - Open a 'File Explorer' window and navigate to ...\Documents\NinjaTrader 8\bin\Custom\ folder. Delete any file that contain Sharkindicators, AWSSDK, Betwixt, Castle.Core, Jil, Ninject, PLUS, or Sigil in the file name. Do Not delete files that have a .lic extension.
(Click image to expand)
- Developer Extensions Note: The SharkIndicators.BloodHound.cs and SharkIndicators.Common.cs files are for Dev. Ext.
- Navigate to …\NinjaTrader 8\bin\Custom\Strategy\ folder. If files exist, delete SiBloodHoundStrategyExample.cs and SiBloodHoundStrategyExample2.cs. And, any other custom strategy that utilizes BloodHound.
- Skip this step if Developer Extensions are Not installed. This step removes Dev. Ext. entries from the Config.xml file. If you are fixing Dev. Ext. that were installed because you have a tool like TDU Bloodhound Statistics or Smart TradeMarkers, you may want to have their support person help you with this step.
Navigate your File Explorer to the ...\Documents\NinjaTrader 8\ folder. - Restart NinjaTrader (without SharkIndicators software).
- Perform a NinjaScript compile test.
- From the Control Center go to New » NinjaScript Editor.
- In the editor window, Right-Click and then select Compile (F5).
- If the NinjaScript editor shows errors...
- If there are no errors, then close NinjaTrader, and reinstall SharkIndicators software again.
- Remove all custom NinjaScript code that references BloodHound. Files such as 'TDU Bloodhound Statistics' or 'Smart TradeMarkers'. All custom strategies and indicators that references or uses BloodHound must be removed/uninstalled from NinjaTrader first.
Microsoft Visual Studio
When compiling your NinjaScript projects directly in Visual Studio, if you plan to reference any of SharkIndicators' .dll's, you will need to first set the target .NET version from the default 4.5 to 4.8.
To do this, right click the NinjaTrader.Custom project in visual studio and select Properties.
Under the Applications tab find the Target Framework and set that to .NET Framework 4.8.
NinjaScript Indicator Tester
This is an indicator "wrapper" that creates the same environment as using an indicator in BloodHound. This wrapper is intented to help indicator developers debug their indicators by visually seeing, on the chart, the data that BloodHound/BlackBird is receiving. NinjaTrader is forgiving when running an indicator on a chart, but is very strict when running an indicator inside another indicator.
Instructions on how to use this wrapper are written within the code. Open this indicator using the NinjaScript Editor to read. Knowledge of NinjaScript programming is required.
Please Note: SharkIndicators does not provide any type of programming help or support in any form. If you need assistance, please contact a NinjaScript programmer, or the author of the indicator you are trying to test, or NinjaTrader support for general NinjaScript help.
Import into NinjaTrader 8 using the standard .zip file import process.
Click to download, IndicatorTesterForBloodHound_NT8.zip